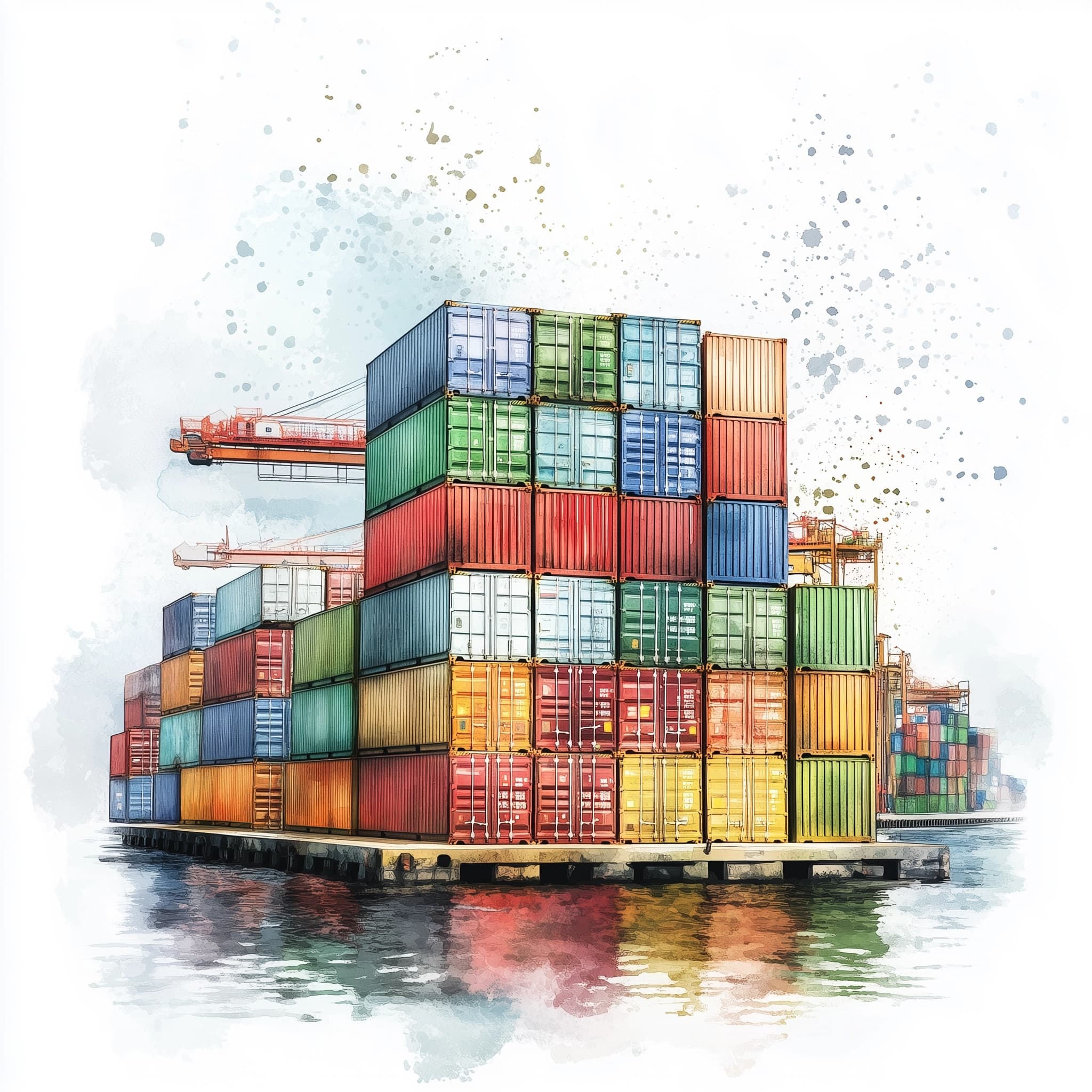
8 Common Python Package Management Mistakes to Avoid
Python package management can make or break your project. Avoiding common mistakes ensures stability, security, and smooth collaboration. Here’s what you need to know to keep your Python projects on track:
-
Skipping Virtual Environments: Leads to dependency conflicts. Use tools like
venv
, Poetry, or Conda to isolate dependencies. -
Using Improper Dependency Versions: Pin exact versions for production; use semantic versioning to manage updates smartly.
-
Overlooking Security in Packages: Regularly audit packages for vulnerabilities using tools like
pip-audit
. -
Not Documenting Dependencies: Maintain clear records with
requirements.txt
orpyproject.toml
for consistent setups. -
Ignoring Transitive Dependencies: Hidden risks from indirect dependencies can be managed with Poetry or
pip-tools
. -
Not Testing Dependencies: Regular testing with CI/CD pipelines and tools like
pip check
prevents issues. -
Not Using a Package Manager Effectively: Leverage pip, Poetry, or Conda based on your project’s complexity.
-
Not Keeping Packages Updated: Outdated dependencies expose your project to security and compatibility risks. Use automated checks to stay current.
Quick Comparison:
Mistake | Risk | Solution |
---|---|---|
Skipping Virtual Environments | Dependency clashes | Use venv , Poetry, or Conda |
Improper Dependency Versions | Project instability | Pin versions, follow semantic versioning |
Overlooking Security | Vulnerabilities | Use pip-audit , review packages |
Not Documenting Dependencies | Setup inconsistencies | Use requirements.txt , pyproject.toml |
Ignoring Transitive Dependencies | Hidden conflicts and risks | Use Poetry, pip-tools |
Not Testing Dependencies | Compatibility issues | Test with CI/CD pipelines |
Ineffective Package Management | Version conflicts, inefficiency | Choose the right tool (pip, Poetry) |
Not Updating Packages | Security flaws, bugs | Automate updates, use pip list --outdated |
Key takeaway: Effective Python package management isn’t just about installing libraries - it’s about creating stable, secure, and maintainable projects. Address these mistakes to save time and avoid unnecessary headaches.
Which Python Package Manager Should You Use?
1. Skipping Virtual Environments
All operating systems come installed with a base version of Python. Most also include a default version of pip
, Python's
official package manager. Installing packages is as simple as pip install package-name
. However, this simplicity can lead to
problems when working on multiple projects. A simple pip install will download these packages globally so they're accessible
throughout your operating system. Perhaps okay if you're only working on a script or two, but when you start working on
multiple projects you'll quickly run into dependency conflicts. This is where virtual environments come in.
A virtual environment isolates your python version and packages, so doing a pip install
will only affect the current
project. These environments keep project dependencies separate, and usually perform more explicit version pinning, ensuring
consistent behavior whether you're in development or production.
Here's a breakdown of how different practices impact project stability:
Approach | Development Challenges | Production Risks |
---|---|---|
Global Installation | Dependencies clash across projects | Environments become inconsistent |
Virtual Environments | Keeps dependencies isolated per project | Builds are reliable and reproducible |
No Version Control | Leads to unpredictable behavior | Increases the risk of deployment failures |
Managed Environments | Provides a consistent experience | Ensures stable deployments |
Tools for Setting Up Virtual Environments
Python offers several tools to help manage virtual environments effectively:
-
venv: A built-in Python tool for creating simple, isolated environments.
-
Poetry: Handles virtual environments while also providing features like dependency management and publishing.
-
Conda: Great for data science projects, as it supports multiple languages and complex dependencies.
When choosing a tool, think about these factors:
-
Project complexity: For basic projects,
venv
is sufficient. For more intricate setups, Poetry or Conda might be better. -
Team expertise: Match the tool to your team's comfort level.
-
CI/CD integration: Make sure the tool works smoothly with your existing CI/CD pipeline.
Once your virtual environment is set up, focus on managing dependency versions to avoid compatibility issues.
2. Using Improper Dependency Versions
Using the wrong dependency versions can cause unexpected project failures, disrupt deployments, and lead to inconsistent behavior across environments. This can jeopardize application stability, slow down team productivity, and even open the door to security risks.
How Semantic Versioning Works
Semantic versioning (e.g., 2.25.1) follows the format MAJOR.MINOR.PATCH to signal breaking changes, new features, and bug fixes. Here's a quick breakdown:
Version Component | Purpose | When to Update |
---|---|---|
MAJOR (2.x.x) | Breaking changes | When changes break backward compatibility |
MINOR (x.25.x) | New features | When adding functionality that doesn't break compatibility |
PATCH (x.x.1) | Bug fixes | For backward-compatible fixes to existing issues |
Managing Version Constraints
For production environments, always pin exact versions (e.g., requests==2.25.1
) to avoid surprises. During development, allow some flexibility with ranges like requests>=2.25,<3.0
to maintain compatibility while enabling updates.
Version Specification | Best Use | Example |
---|---|---|
Exact Pin | Stable production deployments | requests==2.25.1 |
Minor Version Range | Flexible development | requests>=2.25,<3.0 |
Latest Minor | Active development | requests~=2.25 |
Keep dependencies organized in files like requirements.txt
or pyproject.toml
for clarity and easy reproducibility:
[tool.poetry.dependencies]
requests = "^2.25"
numpy = "~=1.20"
pandas = "==1.4.2"
Tips for Better Dependency Management
-
Run
pip check
often to catch version conflicts early. -
Document your versioning decisions to make them easy to track and explain.
-
Test your application with both the minimum and maximum allowed dependency versions.
-
Update dependencies in a systematic way, rather than making random changes.
While proper dependency versioning protects your project from instability, ignoring security risks can be just as damaging. We'll dive into that in the next section.
3. Overlooking Security in Packages
Ignoring security in Python packages can lead to serious problems, including compromised credentials and systems. For private packages, the stakes are even higher - proprietary code and sensitive business data could be exposed.
How to Choose Reliable Packages
When selecting Python packages, focus on these important security factors:
Security Factor | What to Look For |
---|---|
Maintenance | Regular updates and active development; avoid packages with no updates for over 6 months |
Community | A strong group of contributors and responsive issue resolution |
Documentation | Up-to-date, clear documentation and usage instructions |
Download Stats | Steady and reasonable download numbers that align with the package's age and popularity |
To ensure a package is trustworthy, review its source code, the contributors behind it, and its update history.
Tools to Strengthen Package Security
Using the right tools can help safeguard your Python development process:
Tool | Purpose |
---|---|
pip audit | Scans dependencies for known security vulnerabilities |
ProGet | Helps manage package approval workflows |
Envelope | Offers secure hosting for private packages |
For automated vulnerability checks, integrate tools like pip-audit
into your CI/CD pipeline:
# Add this to your CI/CD workflow
pip install pip-audit
pip-audit --requirement requirements.txt
"A package approval workflow is just like a code review but for open-source packages from PyPI." - Inedo Blog
In enterprise setups, consider implementing a structured package approval process that includes:
-
Regular security reviews
-
Controlled access to private packages
-
Automated tools for vulnerability scanning
Securing your packages is essential, but don't forget to document all dependencies. Clear records make it easier for your team to maintain and audit them when needed.
4. Not Documenting Dependencies
Clear and detailed documentation is crucial for managing packages effectively. Without it, projects can quickly become unstable and difficult to maintain, often leading to the dreaded "works on my machine" problem.
Why Dependency Documentation Matters
Properly documenting dependencies keeps your projects stable and your team efficient. A 2023 JetBrains Python Developer Survey found that 78% of teams identified undocumented dependencies as a major source of deployment headaches.
Here’s how documenting dependencies can help:
Benefit | Impact |
---|---|
Reproducibility | Prevents version conflicts and ensures consistent performance. |
Onboarding Efficiency | Speeds up new developer setup, saving hours of time. |
Security Tracking | Makes it easier to identify and update vulnerable packages. |
Using requirements.txt
and pyproject.toml
In Python projects, tools like requirements.txt
and pyproject.toml
are commonly used to document dependencies. For a quick snapshot of your current dependencies, you can use pip freeze
to generate a requirements.txt
file.
For more advanced needs, pyproject.toml
offers additional features, such as improved dependency resolution and project metadata management:
[project]
dependencies = ["requests>=2.28.0", "numpy>=1.23.0,<2.0.0"]
To ensure consistency, stick to clear version specifications and establish team-wide practices for documenting dependencies. Regularly reviewing and updating these files will help avoid mismatches between development and production environments.
While documenting direct dependencies is essential, don’t overlook transitive dependencies - they can introduce hidden problems, which we’ll explore in the next section.
5. Ignoring Transitive Dependencies
Documenting direct dependencies is helpful, but overlooking transitive dependencies can create hidden risks. These indirect dependencies can affect your project's stability and security if left unchecked.
What Are Transitive Dependencies?
Transitive dependencies are the indirect libraries your project inherits through its direct dependencies. For example, if you're using the requests
library, it also brings in dependencies like urllib3
and certifi
. While these additional libraries run behind the scenes, they can lead to issues like version conflicts, security vulnerabilities, or unnecessary project bloat.
Here are some common problems caused by transitive dependencies:
-
Conflicts when different packages require incompatible versions of the same library
-
Potential security flaws (refer to Section 3 for more details on securing dependencies)
-
Extra, unneeded dependencies that make your project larger and harder to manage
Tools to Handle Transitive Dependencies
Fortunately, modern Python tools can help you manage these indirect dependencies with ease:
Poetry's Dependency Resolution
Poetry simplifies dependency management by automatically resolving transitive dependencies. Its pyproject.toml
file ensures consistency across team environments:
[tool.poetry.dependencies]
python = "^3.8"
requests = "^2.28.0"
pip-tools for Detailed Control
If you need more granular control, pip-tools
can generate a comprehensive requirements.txt
file with all dependencies and their exact versions:
pip-compile pyproject.toml --output-file requirements.txt
Best Practices for Managing Transitive Dependencies
To keep your Python environment stable and secure:
-
Regularly review and resolve dependency conflicts using tools like Poetry
-
Include automated dependency checks in your CI/CD pipeline
-
Update libraries systematically to avoid technical debt and security risks
6. Not Testing Dependencies
Managing transitive dependencies helps reduce hidden risks, but testing all dependencies is just as important. Regular testing ensures your project stays stable and secure by catching compatibility issues before they hit production.
Why Dependency Testing Matters
Testing dependencies regularly helps you spot version conflicts, security flaws, and compatibility issues early. This proactive approach keeps your production environment safe and smooth.
Here’s what dependency testing can do for you:
-
Improved Security: Detects vulnerabilities in your dependencies.
-
Better Performance: Confirms that dependencies work well together without causing slowdowns.
-
Reduced Downtime: Identifies problems during development, saving you from costly production issues.
For a hands-on approach, tools like pip check
can help verify package compatibility:
pip install -r requirements.txt
pip check
Automating Dependency Testing with CI/CD
Automating dependency testing through CI/CD pipelines ensures consistent checks throughout your development process. Here’s an example workflow you can use:
name: Dependency Test
on: [push, pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- name: Check dependencies
run: |
pip install -r requirements.txt
pip check
Tips to Strengthen Your Testing Strategy
-
Test your dependencies weekly across different Python versions to catch issues early.
-
Integrate dependency tests into your broader suite of integration tests.
For larger projects, consider using specialized tools to deepen your testing efforts:
Testing Level | Tool | Purpose |
---|---|---|
Deep Analysis | pip-audit | Thorough dependency review |
Version Compatibility | Tox | Test dependencies across Python versions |
7. Not Using a Package Manager Effectively
Making the most of package managers can help you avoid headaches like version conflicts and security risks.
Popular Package Managers at a Glance
Different package managers are suited for different types of projects:
Package Manager | Best For | Key Features |
---|---|---|
pip | Simple projects | Standard installer, basic dependency handling |
conda | Data science | Manages non-Python dependencies, supports environment management |
poetry | Complex projects | Advanced dependency resolution, lock files, and packaging tools |
Tools like poetry offer features like automatic virtual environment management and precise dependency tracking. Meanwhile, utilities such as pip-tools
enhance what pip can do, offering more control for basic setups.
Choosing the Right Package Manager
Your decision should be guided by your project's needs:
Factor | Consider When | Example Scenario |
---|---|---|
Project Scale | Small vs. Large | Use pip for small scripts; poetry for larger applications |
Team Size | Solo vs. Team | Conda works well for data science teams; poetry suits software development teams |
Dependency Complexity | Simple vs. Complex | Pip handles basic needs; poetry excels with intricate dependency trees |
For corporate settings, consider these strategies:
-
Automate dependency updates to save time
-
Set up private package repositories for internal use
-
Use tools that visualize dependencies to resolve conflicts
-
Explore enterprise solutions like Envelope for hosting private packages
Your choice of package manager can streamline your workflow. For instance, pip works for straightforward projects, but poetry's advanced features shine when dealing with larger, more complex applications. Tools like dependency graphs can also help you spot and fix conflicts, making development smoother.
Keeping dependencies up-to-date is just as important as picking the right package manager. Regular updates ensure your project remains stable and secure.
8. Not Keeping Packages Updated
Skipping package updates is a common oversight in Python package management. Unfortunately, this can lead to avoidable problems, including security risks and compatibility issues. Since PyPI doesn't automatically check for vulnerabilities, it's up to you to keep your dependencies up to date.
"Keeping dependencies up to date is crucial for the security and stability of your project." - GeeksforGeeks
Why You Should Update Packages
Outdated packages can leave your project exposed to security flaws and create compatibility headaches as dependencies evolve. Updating regularly helps maintain stability and keeps your code in sync with the Python ecosystem. Here's a breakdown of why updates are essential:
Update Type | Importance | Risks of Not Updating |
---|---|---|
Security Patches | High | Vulnerabilities and potential exploits |
Bug Fixes | High | Runtime errors and performance problems |
Compatibility Updates | Moderate | Breaking changes and deprecated features |
Keeping your packages current prevents unnecessary technical debt and ensures your project remains secure and functional.
Tools to Help You Stay Updated
To manage updates effectively, use tools like pip
(pip list --outdated
) or poetry
(poetry update
) to identify outdated packages. For locking dependencies, tools like pip-tools
(pip-compile --upgrade
) are invaluable. Automating checks in your CI/CD pipeline can streamline the process, while enterprise tools like Envelope provide more control for private packages.
Here’s how to create an effective update strategy:
-
Automate regular update checks.
-
Test updates in isolated environments (as explained in Section 6).
-
Use version constraints to avoid breaking changes.
Conclusion
Key Takeaways
Managing Python packages effectively requires isolating dependencies, keeping packages current, and thoroughly documenting them. This article highlighted eight common mistakes that can compromise project stability, security, and maintainability. By addressing these issues, teams can create more dependable Python applications while prioritizing security.
Python provides various tools to streamline package management. Here are a few that can help tackle the challenges discussed:
Tool | Purpose | Advantage |
---|---|---|
Poetry | Dependency management | Simplifies environments and updates |
pip-tools | Requirements management | Ensures consistent dependencies |
pip-audit | Security scanning | Detects vulnerabilities |
"A package approval workflow is just like a code review but for open-source packages from PyPI." - Inedo Blog
For enterprise use, tools like Envelope offer secure storage and detailed access controls for private packages, making them ideal for teams handling proprietary code.
To keep your Python package ecosystem in good shape:
-
Automate dependency checks and security scans to save time and mitigate risks.
-
Document all dependencies clearly.
-
Use semantic versioning to handle updates with precision.
FAQs
Here are answers to some common questions about Python package management to make your workflow smoother:
Can PyPI packages be private?
Although PyPI is public, you can create private repositories using manual tools like PyPiServer or hosted platforms such as Envelope. These options allow for secure hosting, access controls, and ample storage.
How does Python resolve dependencies?
Python uses package managers like pip, Poetry, and pip-tools to handle dependencies and ensure compatibility. These tools help identify and fix conflicts, ensuring stable builds.
Tool | Resolution Method | Key Benefit |
---|---|---|
pip | Uses pip check | Flags version conflicts |
Poetry | Leverages poetry.lock | Keeps builds consistent |
pip-tools | Generates requirements.txt | Creates reproducible environments |
Using tools like Poetry and pip-tools can simplify dependency management and keep your environment stable.
Does pip install install all dependencies?
Yes, pip install
automatically pulls in all required dependencies listed in files like setup.py
or pyproject.toml
. To avoid issues, define your dependency tree in a requirements.txt
or pyproject.toml
file for consistent installations across environments.
Easy, private Python packages.
Stop worrying about authorizing git clones, manual indexes, or expensive solutions. Envelope makes hosting private packages as easy as publishing to pypi.
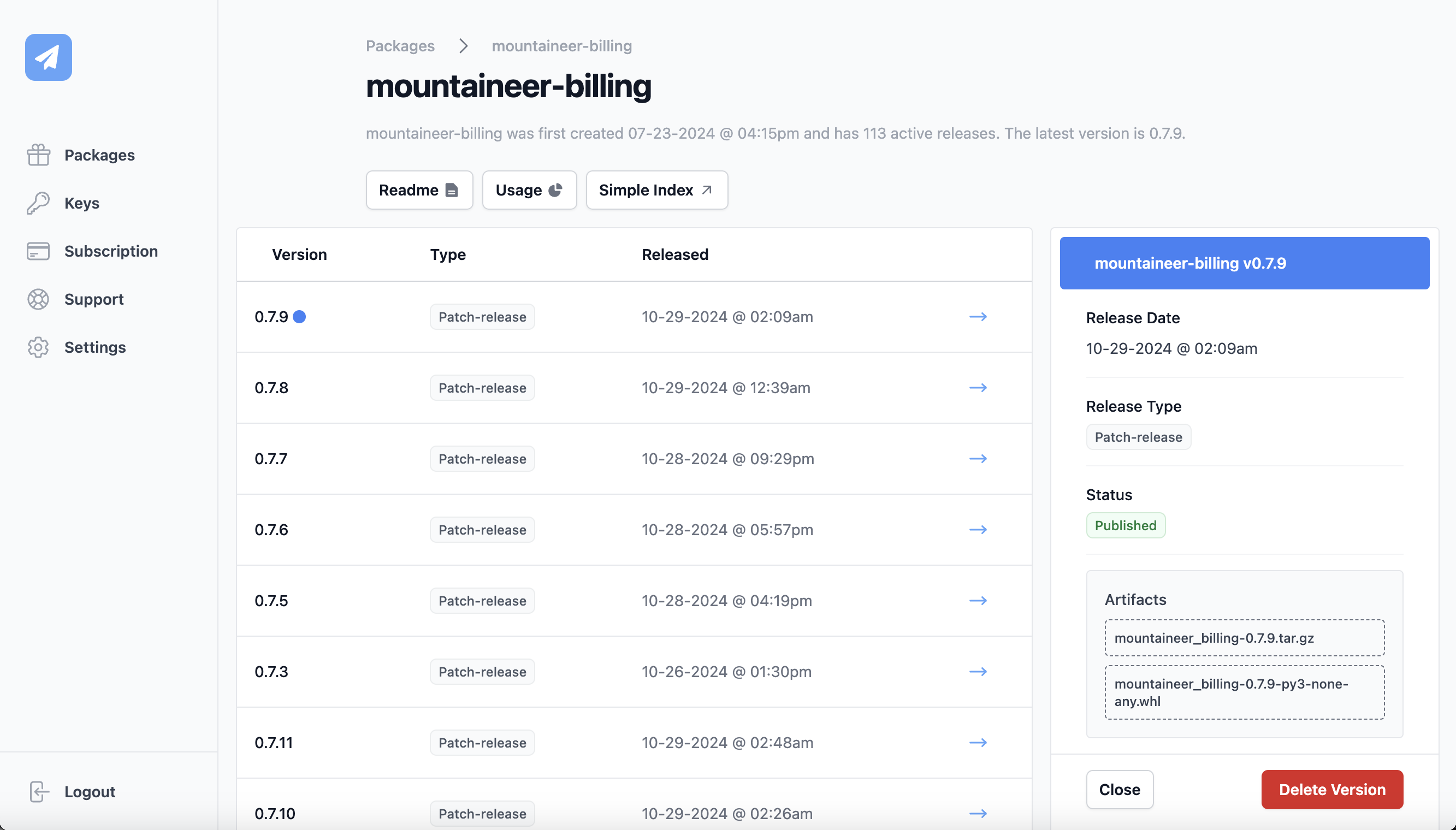